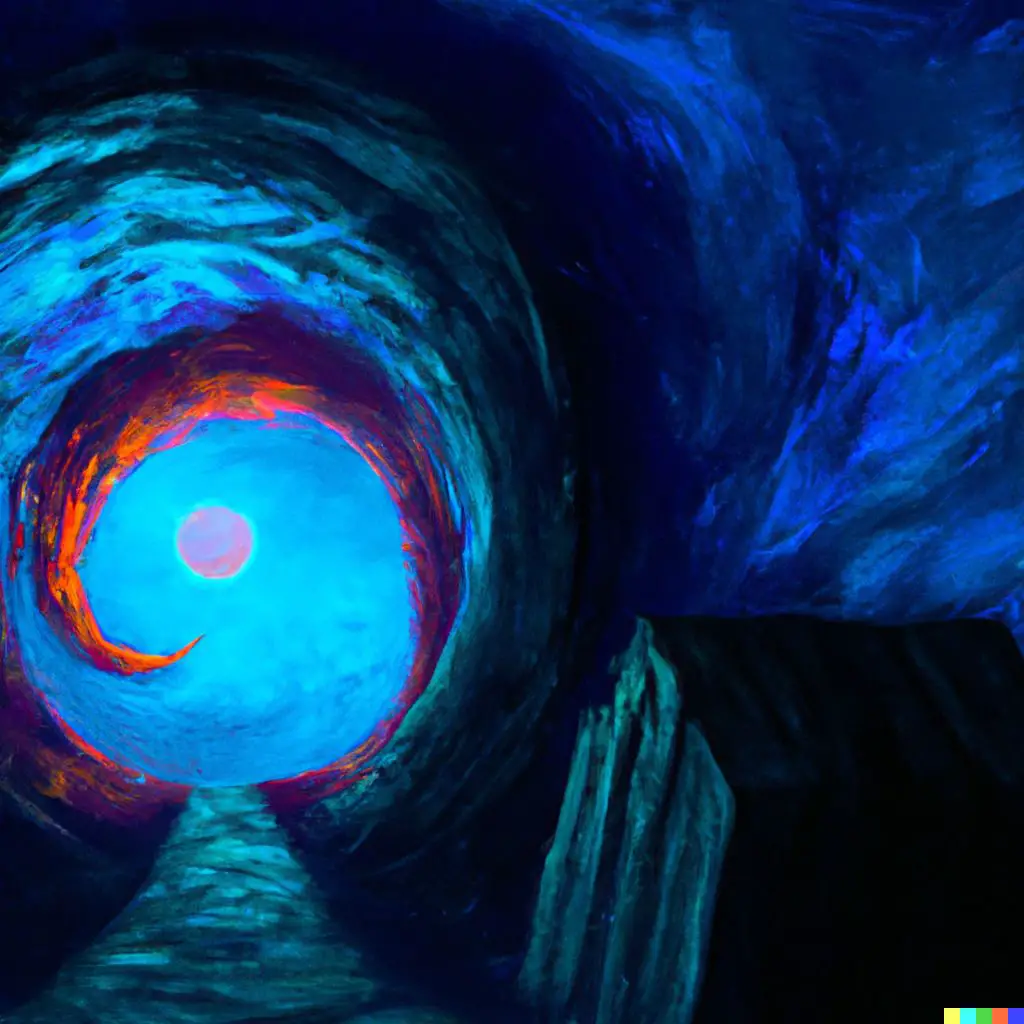
Redirect to an external URL
You can do that in plain JavaScript by calling window.location.replace()
method. For example:
window.location.replace('https://codefrontend.com');
Here's how you could use that in React:
function RedirectExample() {
useEffect(() => {
const timeout = setTimeout(() => {
// 👇️ redirects to an external URL
window.location.replace('https://codefrontend.com');
}, 3000);
return () => clearTimeout(timeout);
}, []);
return <>Will redirect in 3 seconds...</>;
}
window.location.replace
in React.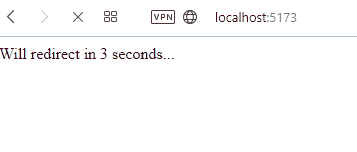
replace()
method, the page will not be accessible via the browser's back button. Real quick - would you like to master Web Development? If so, be sure to check out my recommended platform to learn coding online:
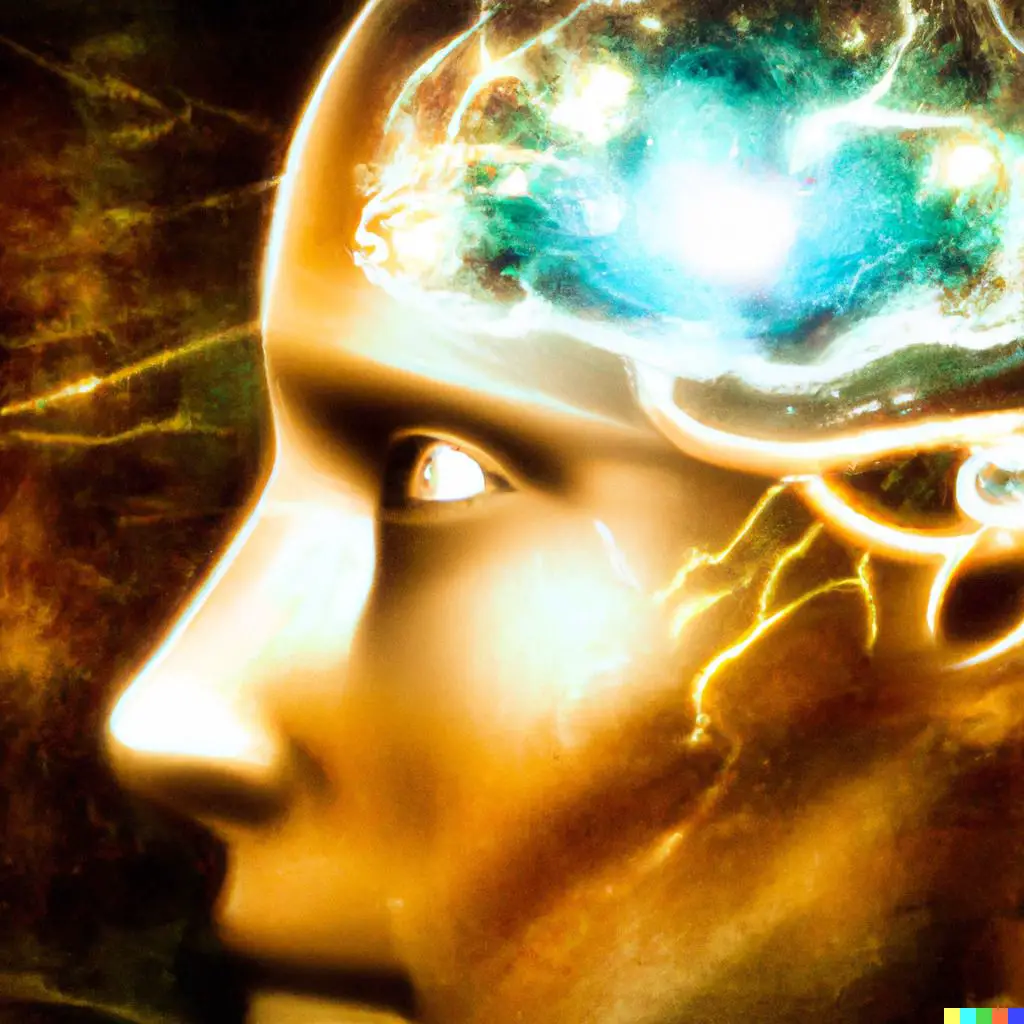
Navigating to an external page in React
Often, by "redirect" people actually mean "navigate.
To navigate to another page, set the window.location.href
property with the URL:
// 👇️ directly change the active URL to navigate
window.location.href = 'https://codefrontend.com';
Navigating like this will add a new entry in the navigation history, instead of replacing the current one, so the user will be able to go back. That's often the expected behavior.
If the navigation happens in response to the user clicking an element, it's often sufficient and better to simply use an anchor tag:
// 👇️ A simple link to an external website
<a href="https://codefrontend.com" target="_blank" rel="noopener noreferrer">
Go to codefrontend.com
</a>
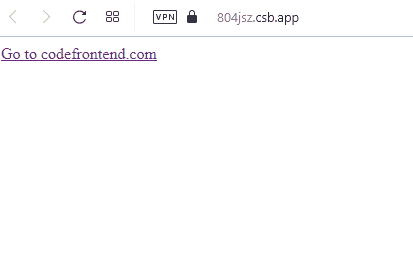
The target="_blank"
attribute can be used to open the link in the new browser tab, remove it to open in the same tab. The rel="noopener noreferrer"
attribute should be added for security reasons. Read more about it here:
Redirect using react-router
It is common to use react-router together with React. It is only responsible for your client-side navigation, meaning that it doesn't handle redirects to external URLs. If you need that, see the above methods.
However, if you need to redirect between the pages in your React app that uses react-router (version 6), you can use the useNavigate hook or Navigate component and set the replace
property to true
: navigate('/about', { replace: true });
Here's a snippet of how it might look like in React:
import { useEffect } from 'react';
import { Route, Routes, useNavigate } from 'react-router-dom';
function RedirectReactRouterExample() {
return (
<Routes>
<Route path="/" element={<Index />} />
<Route path="about" element={<About />} />
</Routes>
);
}
function About() {
return <div>About</div>;
}
function Index() {
const navigate = useNavigate();
useEffect(() => {
setTimeout(() => {
// 👇 Redirects to about page, note the `replace: true`
navigate('/about', { replace: true });
}, 3000);
}, []);
return <div>Redirecting...</div>;
}
export default RedirectReactRouterExample;
Conclusion
Normally, redirects should be done by the server, not the client. However, there are cases when it's needed. In those cases, you can use a simple window.location.redirect()
call.
However, more often than not, what people need is to simply navigate to another page when a button is clicked. In that case, you should set window.location.href
with the target URL, or better yet - use an anchor tag.
You can find the code examples in my GitHub repository.
Hey, if you're serious about learning React, I highly recommend the courses on educative.io - it's my favorite platform for learning to code.
Here's a course on React that will teach you how to build something more than another to-do app (finally, am I right?):
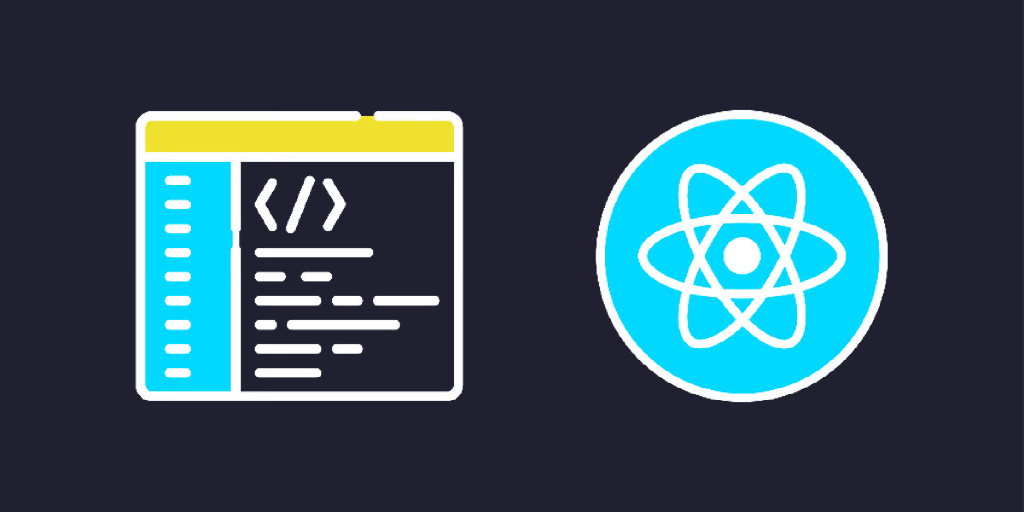