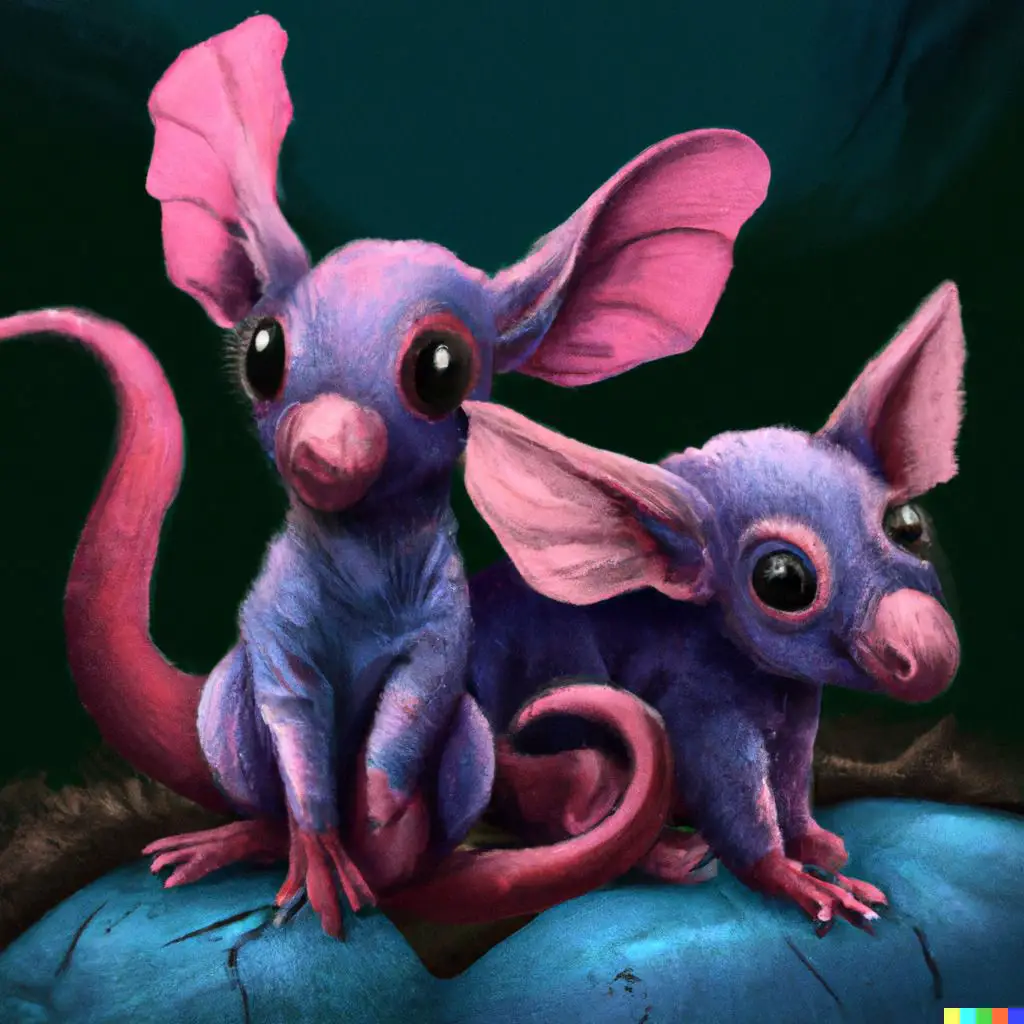
Deduplicate an array of strings or numbers
To remove duplicates in an array of primitive values, such as string or number, you can convert it into a Set and convert it back into an array:
// 👇 Deduplicates an array of primitive values
function deduplicate<T>(array: T[]) {
return Array.from(new Set(array).values());
}
This works because the Set
can only contain unique values. However, it will not work with more complex values such as objects.
Remove duplicates from an array of objects
To deduplicate an array of objects in JavaScript, you should have a way to get a unique string for each item, such as an id
. Then you can store those ids in an object, as you filter the array, and if there's already an id stored, skip the element.
Here's a function to deduplicate an array of objects:
// 👇 Deduplicates array of object values. Determines uniqueness by comparing keys
function deduplicate<T>(array: T[], getKey: (item: T) => string | number) {
const seenItems: Record<string, boolean> = {};
return array.filter((item) => {
const key = getKey(item);
if (seenItems[key]) {
return false;
}
seenItems[key] = true;
return true;
});
}
The function accepts a getKey
function to get a unique string key for each array item. That key will then be used to check uniqueness.
Here's how to use it:
deduplicate(items, (item) => item.id));
id
.Find this and more code examples in my GitHub repository.