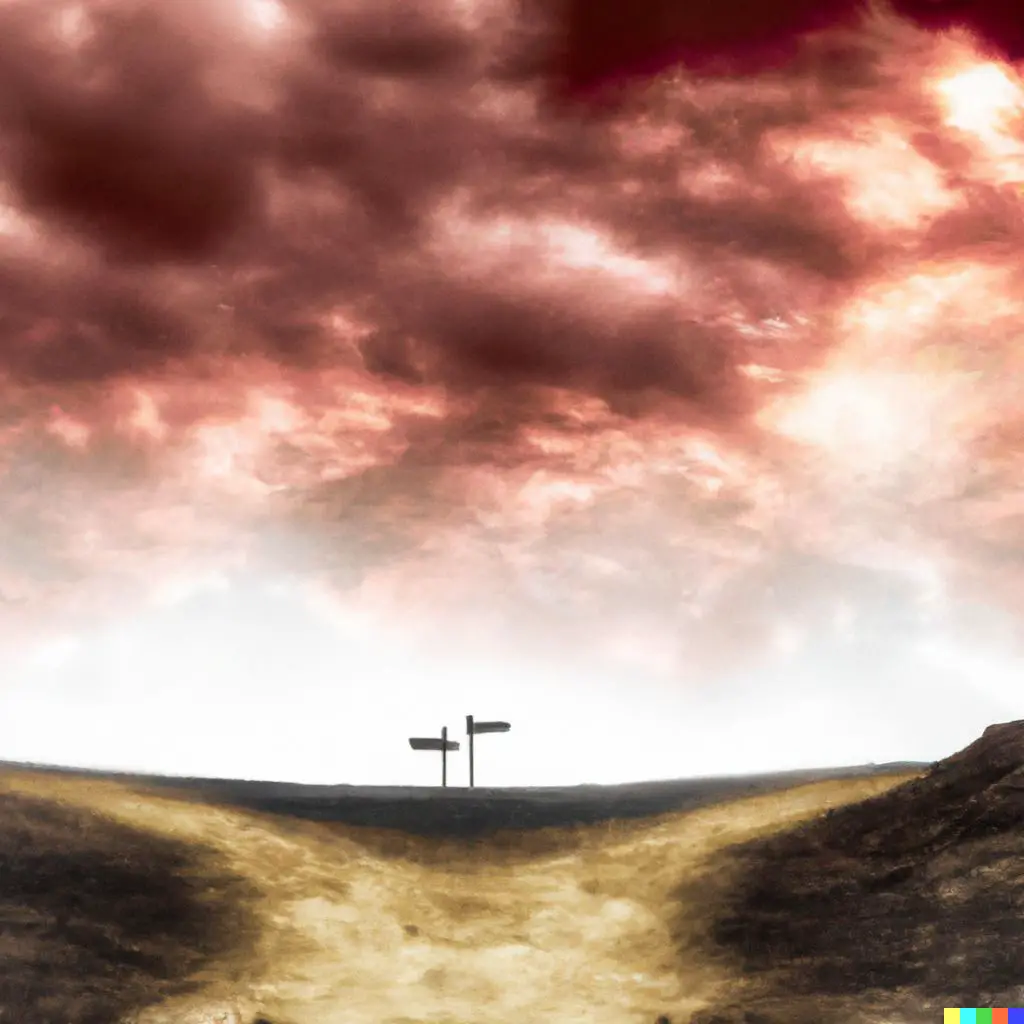
A CSS class can be conditionally applied in React using plain JavaScript:
- Put all CSS classes into an array.
- Use a ternary operator to set an empty string as a class when the condition is
false
. - Join the array using spaces, to convert it into a
className
.
Here's how it's done:
// 👇 Apply the class name on condition
const className = ['bordered accent', isSelected ? 'selected' : ""].join(' ')
// Result: "bordered accent selected" if true
// or "bordered accent " if false
A utility function to join classes
There are two ways we can improve on the basic approach:
- Using the JavaScript
&&
operator to set classes only on truthy values. - Filter out the falsy values from className array before joining them.
We can write a reusable function we can use to generate the `className` string with the above improvements. Here it is:
function classNames(...args: any[]) {
return args.filter(Boolean).join(' ')
}
: any[]
part from the function parameters.The classNames
utility function can be used to apply the classes conditionally in React like so:
import { useState } from 'react';
import classNames from './classNames';
import './style.css';
function Example() {
const [isSelected, setSelected] = useState(false);
return (
<button
className={classNames('bordered accent', isSelected && 'selected')}
onClick={() => setSelected(!isSelected)}
>
Click Me
</button>
);
}
classNames
utility function to apply classes conditionally in ReactHere's how the CSS classes look like in style.css
:
.bordered {
border: 1px dashed black;
}
.accent {
background-color: lightblue;
}
.selected {
background-color: blue;
color: white;
}
This is what it looks like when we click the button:
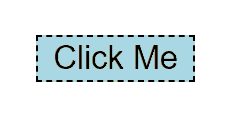
selected
class is applied conditionally onClick Conclusion
Conditionally applying classes in React is no different than doing the same with plain JavaScript. It's only a matter of joining them into a single string, with classes separated by spaces.
Feel free to use the classNames
utility I've shown to help join the classes into the string. Then, to conditionally apply the class, simply use &&
operator after the condition.
Find the full example code in my GitHub repo.
Hey, if you're serious about learning React, I highly recommend the courses on educative.io - it's my favorite platform for learning to code.
Here's a course on React that will teach you how to build something more than another to-do app (finally, am I right?):
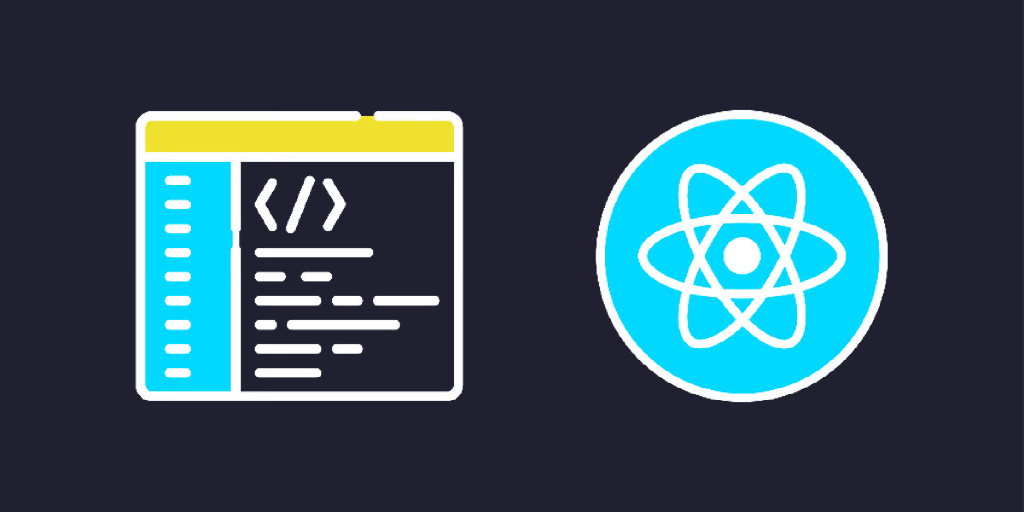