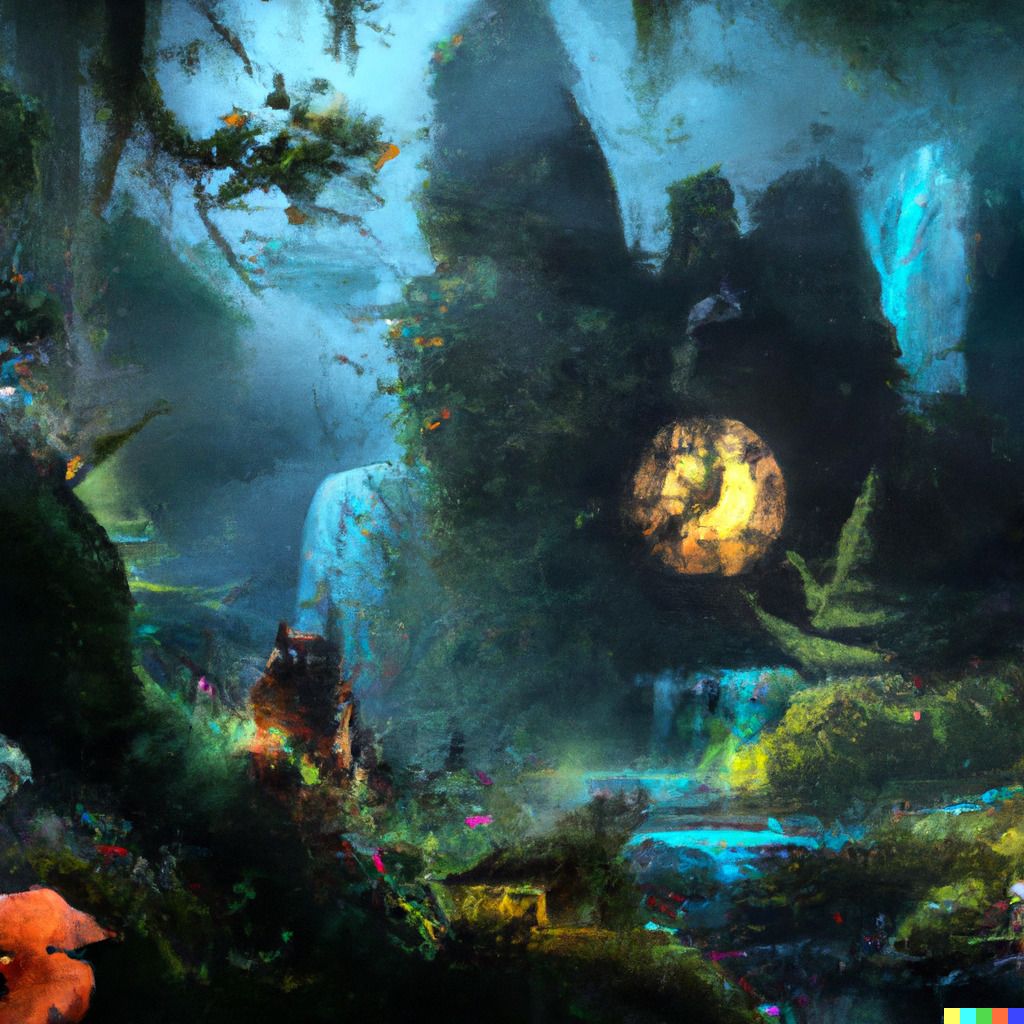
Timestamp in milliseconds
Use the static function Date.now() to get a timestamp in JavaScript:
// 👇 Current timestamp in milliseconds
function getCurrentTimestamp() {
return Date.now();
}
The timestamp is milliseconds since January 1, 1970 in UTC. The Date.now()
function in JS returns the timestamp of the current time.
Timestamp from a date string
If you already have a Date
object, you can use the prototype methods getTime() or valueOf() to convert it to timestamp:
const exactDate = '09/19/2022 10:58:13';
// 👇 Get the timestamp when you already have the date object
const exactDateTimestamp = new Date(exactDate).getTime();
console.log(exactDateTimestamp); // 1663574293000
- Create a new
Date
object from a date string by using the constructor. - Call the
getTime()
function on theDate
object to get the timestamp in milliseconds. - Divide the result by
1000
and round it down to get a Unix timestamp.
The getTime()
and valueOf()
functions do the exact same thing and can be used interchangeably.
privacy.reduceTimerPrecision
or privacy.resistFingerprinting
, your result may be rounded, and not come in milliseconds. If that can cause a problem for you, consider implementing your own checks for that. Read more on MDN.Unix timestamp
The Unix timestamp is in seconds rather than milliseconds. Simply divide the timestamp by 1000:
// 👇 To get UNIX timestamp, divide by 1000
function getCurrentUnixTimestamp() {
return Math.floor(Date.now() / 1000);
}
const exactDate = '09/19/2022 10:58:13';
// 👇 Get the timestamp when you already have the date object
const exactDateTimestamp = new Date(exactDate).getTime();
console.log(exactDateTimestamp); // 1663574293
Conclusion
Javascript has native utils to get a timestamp in milliseconds. However, manipulating dates is notoriously complicated. If you need to do more complex date calculations I recommend using a purpose-built date manipulation library date-fns.
Incidentally, date-fns has functions to get the timestamps, both regular and Unix. In addition, it has functions to format and localize dates, and do date maths.
You can find the code examples in my GitHub repo.
Hey, if you're serious about learning JavaScript, I highly recommend the courses on educative.io - it's my favorite platform for learning to code online.
Here's a course on JavaScript that will teach you everything you need to know:
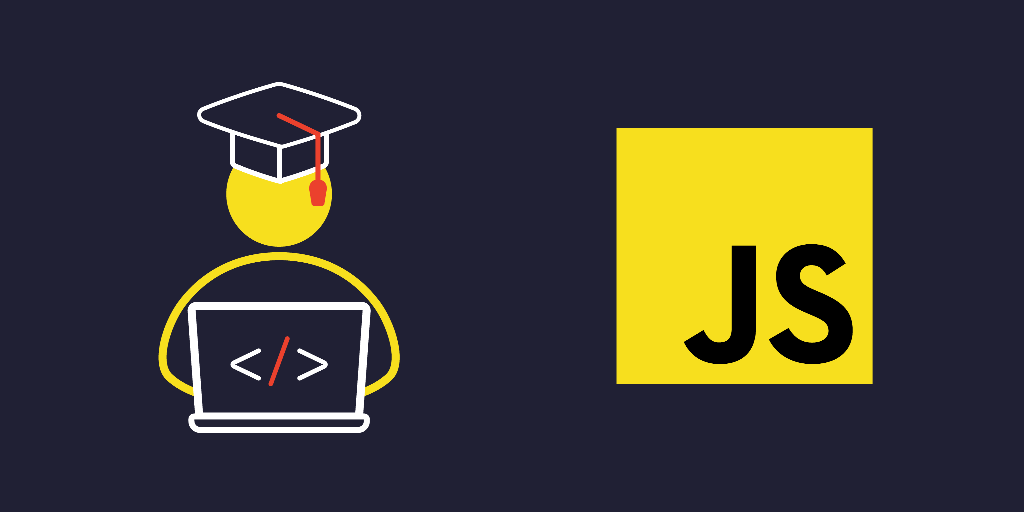